What is spiral order matrices
We have to print the given 2D matrix in the spiral order. Spiral Order means that firstly, first row is printed, then last column is printed,
then last row is printed and then first column is printed, then we will come.
Example-
1 | 5 | 7 | 9 | 10 | 11 |
6 | 10 | 12 | 13 | 20 | 21 |
9 | 25 | 29 | 30 | 32 | 41 |
15 | 55 | 59 | 63 | 68 | 70 |
40 | 70 | 79 | 81 | 95 | 105 |
Output
Output of the example like or it will print like this
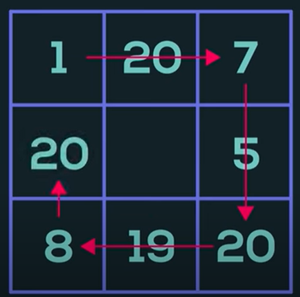
Algorithm
Spiral order is given by:
1 5 7 9 10 11 21 41 70 105 95 81 79 70 40 15 9 6 10 12 13 20 32 68 63 59 55 25 29 30 29.
(We are given 2D matrix of n X m ).
1. We will need 4 variables:
a. row_start - initialized with 0.
b. row_end - initialized with n-1.
c. column_start - initialized with 0.
d. column_end - initialized with m-1.
2. First of all, we will traverse in the row row_start from column_start
to column_end and we will increase the row_start with 1 as we have
traversed the starting row.
3. Then we will traverse in the column column_end from row_start to
row_end and decrease the column_end by 1.
4. Then we will traverse in the row row_end from column_end to
column_start and decrease the row_end by 1.
5. Then we will traverse in the column column_start from row_end to
row_start and increase the column_start by 1.
6. We will do the above steps from 2 to 5 until row_start <= row_end
and column_start <= column_end.
Coding
#include <bits/stdc++.h> using namespace std; int main() { int row, col; cout << "Enter the size of row :"; cin >> row; cout << "Enter the size of col :"; cin >> col; int arr[row][col]; cout << "\nEnter the elements \n"; for (int i = 0; i < row; i++) { for (int j = 0; j < col; j++) { cin >> arr[i][j]; } } // Sprial order logic int rowstart = 0, rowend = row - 1, colstart = 0, colend = col - 1; while (rowstart <= rowend && colstart <= colend) { // For row start for (int column = colstart; column <= colend; column++) cout << arr[rowstart][column] << " "; rowstart++; // For col end for (int rows = rowstart; rows <= rowend; rows++) cout << arr[rows][colend] << " "; colend--; // For row end for (int column = colend; column >= colstart; column--) cout << arr[rowend][column] << " "; rowend--; // For column start for (int rows = rowend; rows >= rowstart; rows--) cout << arr[rows][colstart]<<" "; colstart++; } return 0; }
Output
Enter the size of row :5 Enter the size of col :6 Enter the elements 1 5 7 9 10 11 6 10 12 13 20 21 9 25 29 30 32 41 15 55 59 63 68 70 40 70 79 81 95 105 1 5 7 9 10 11 21 41 70 105 95 81 79 70 40 15 9 6 10 12 13 20 32 68 63 59 55 25 29 30 29
Check Out OUR Home page for more information about practice problemAlso check Out
Also check Out Projects for Resume
Problems On C++Q-2) Vectors In c++Problems on TCS paper
1 , 1 , 2 , 3 , 4 , 9 , 8 , 27 , 16 , 81 , 32 , 243 , 64 , 729 , 128 , 2187
0 , 0 , 2 , 1 , 4 , 2 , 6 , 3 , 8 , 4 , 10 , 5 , 12 , 6 , 14 , 7 , 16 , 8
FEEDBACK
If still you have any doubts please comment below and please share your approaches with us .
Post a Comment
Post a Comment
Please do not spam in comment